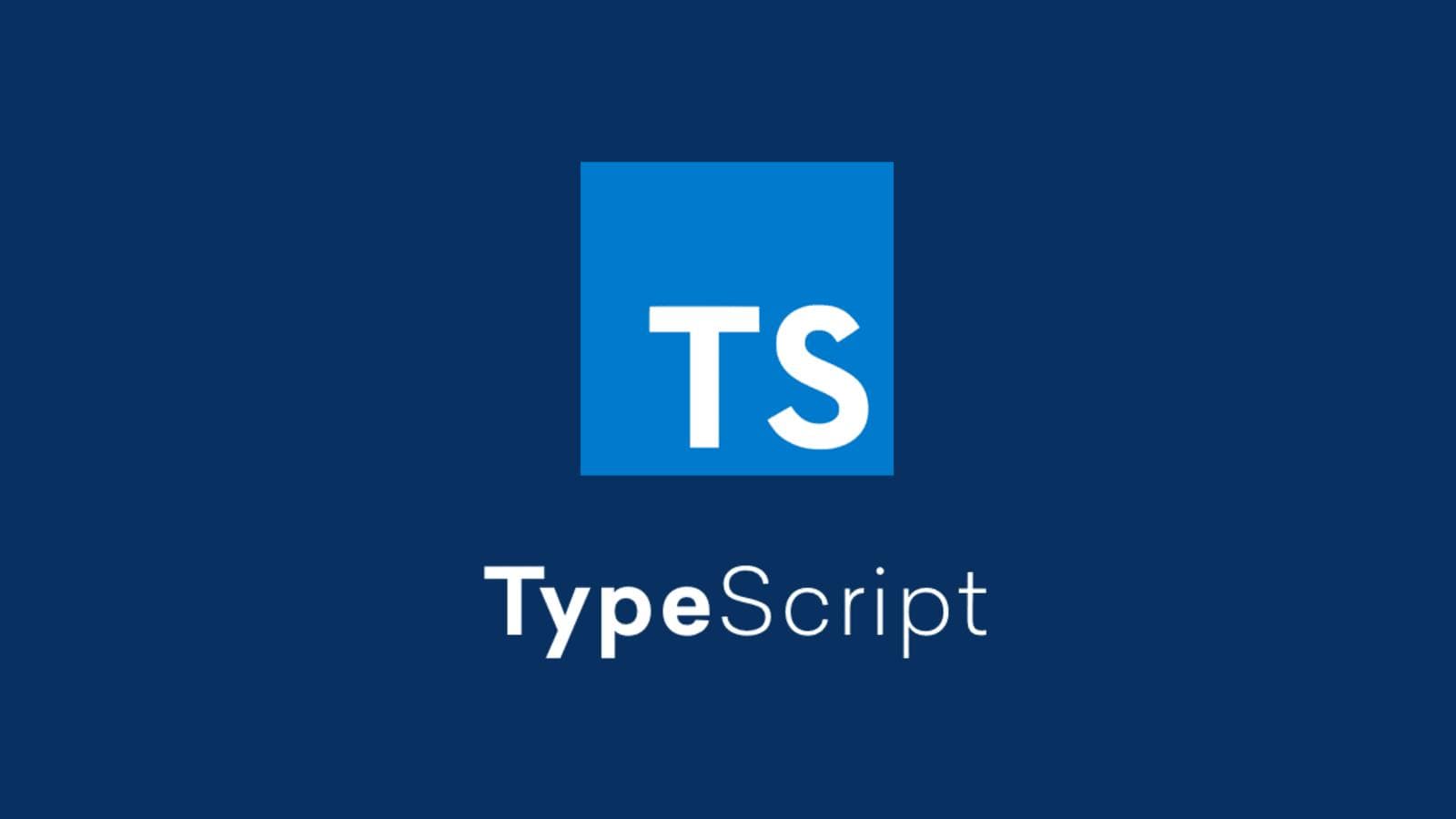
Introduction:
TypeScript has rapidly gained popularity among developers as a powerful tool for building robust and maintainable software. By adding static typing and other advanced features to JavaScript, TypeScript enhances developer productivity, code quality, and overall project maintainability. In this comprehensive guide, we'll explore the various aspects of TypeScript and demonstrate how it empowers developers to write safer, more scalable, and efficient code.
Understanding TypeScript:
TypeScript is a statically typed superset of JavaScript that compiles down to plain JavaScript. It introduces a type system, allowing developers to define types for variables, function parameters, return values, and more. This static typing helps catch errors early in the development process and provides better tooling support, such as code editors' autocomplete and type checking features.
Getting Started with TypeScript:
To get started with TypeScript, you'll need to install it globally or locally in your project using npm or yarn. Once installed, you can initialize a new TypeScript project using the tsc --init command, which generates a tsconfig.json file. This file configures TypeScript compiler options for your project, such as target ECMAScript version, module system, and more.
Basic Types and Type Inference:
TypeScript supports various basic types such as number, string, boolean, object, array, tuple, enum, any, and more. You can also create custom types using interfaces, type aliases, and classes. TypeScript's type inference feature automatically infers types based on the context, reducing the need for explicit type annotations.
Example:
// Type inference
let num: number = 5; // TypeScript infers the type as number
let message = "Hello, TypeScript!"; // TypeScript infers the type as string
// Custom type using interface
interface Person {
name: string;
age: number;
}
let person: Person = { name: "Alice", age: 30 };
Advanced TypeScript Features:
TypeScript offers a wide range of advanced features such as union and intersection types, generics, type guards, conditional types, keyof operator, mapped types, and more. These features enable developers to express complex relationships between types and create highly reusable and flexible code.
Example:
// Union types
type Result = number | string;
function processResult(result: Result): void {
if (typeof result === "number") {
console.log("Numeric result:", result);
} else {
console.log("String result:", result);
}
}
Integrating TypeScript with Modern JavaScript Frameworks:
TypeScript is widely adopted in modern JavaScript frameworks such as React, Angular, Vue.js, and Node.js. It provides enhanced tooling support, better type safety, and improved developer experience when building applications with these frameworks. TypeScript's integration with popular libraries and frameworks enables developers to leverage the full power of static typing in their projects.
Example (React):
// TypeScript in React
import React, { useState } from 'react';
interface CounterProps {
initialValue: number;
}
const Counter: React.FC<CounterProps> = ({ initialValue }) => {
const [count, setCount] = useState<number>(initialValue);
const increment = () => setCount(count + 1);
const decrement = () => setCount(count - 1);
return (
<div>
<button onClick={increment}>Increment</button>
<button onClick={decrement}>Decrement</button>
<p>Count: {count}</p>
</div>
);
};
Conclusion:
TypeScript offers a powerful set of features that elevate JavaScript development to the next level. By embracing TypeScript, developers can write safer, more maintainable, and scalable code, ultimately improving the overall software quality and developer productivity. Whether you're building web applications, server-side APIs, or large-scale enterprise systems, TypeScript empowers you to tackle complex challenges with confidence and efficiency. Embrace the power of TypeScript and unlock new possibilities in modern software development.

Osaretin Igbinobaro
Software Engineer @ Apadmi